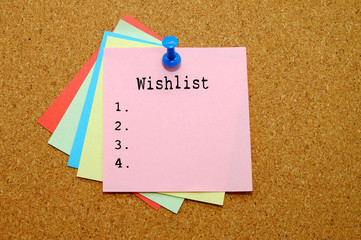
YITH Wishlist Plugin is one of the most popular plugin for Woocommerce to add a Wishlist to your WordPress website. The good thing about this plugin is they make it very simple to add the wishlist button to the product page. If you are a regular user, there isn’t many things else to do. However, if you are a developer, you may wonder how can you get the wishlist URL so you can integrate to your own plugin or theme. That’s exactly what I’m going to show you in this post.
How to get the add to wishlist URL of a particular product
If you need nothing else but a working add to wishlist button, you can put this in your code:
do_shortcode('[yith_wcwl_add_to_wishlist product_id=your_product_id]');
Make sure you replace your_product_id with actual ID.
However, YITH wishlist plugin only provide you with the text link like this:
What if you want to remove the text and get just the heart?
Well, this is how you can get only the URL of the add to wishlist button of YITH wishlist plugin:
esc_url( add_query_arg( 'add_to_wishlist', $your_product_id ) )
What you get from this code is an URL. When you go to that URL, the product will be added to their wishlist.
Pretty easy, isn’t it.
However, I would recommend you send an ajax request to that url instead of open it. That would improve the visitors’ experience greatly.
Caution
One final warning to plugin developers and users of wishlist plugin alike, don’t use multiple wishlist plugins on your site. The reason is because, for example, you installed and activate two wishlist plugin called A and B. There are times you add a product to A’s wishlist and try to view the B’s wishlist page. You will see things that you may not expected. So, remember not to use two different wishlist plugin on one site.